Chapter 5. Object identity
Each object has its own identity
Important
- Object Identity
is the property by which each object (regardless of its class or current state) can be identified and treated as a distinct software entity.
There is "something unique" about any object that distinguishes it from other objects. This unique thing is the object-handle (sometimes calles object reference).
Example of an object creation (books notation):
var hom1: Hominoid := Hominoid.New;
Example of an object creation (C++ notation):
Hominoid *hom1 = new Hominoid();
The right hand side creates a new object of class Hominoid, and returns an object handle. In the case of this example the handle is 602237.
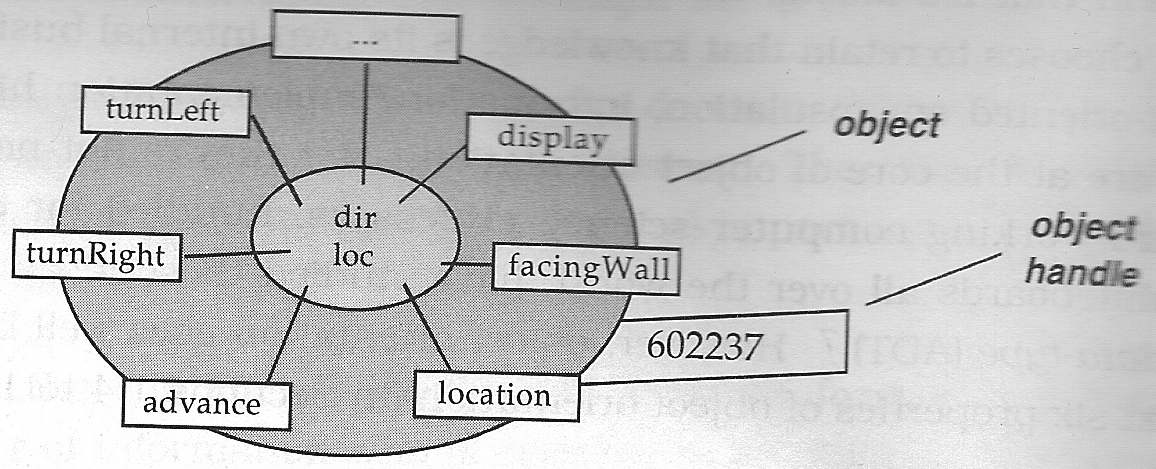
Two rules apply for handles:
The same handle stays with the object for all time!
-
No two objects can have the same handle. Every new object gets its own handle that is different. Objects may look identical (same state, same class) but if they have different handles they are different objects! If they have the same handle they are the same.
In most OO languages (Java for example) handles are different from all existing or having existed objects. In C++ handles can be re-used if the original object is dead.
C++ uses the memory address as object handle.
The left part of the line ( var hom1:Hominoid / Homoid *hom1 ) is a declaration that gives a programmer-meaningful name (hom1) to a space that can hold an object handle.
The assignment ( := / = ) causes hom1 to hold the handle to the object. Read: "now points to" or "now refers to"
In the case above: hom1 now referes to a new object of class Hominoid.
Usually you don't see the handle, but just use the variables.
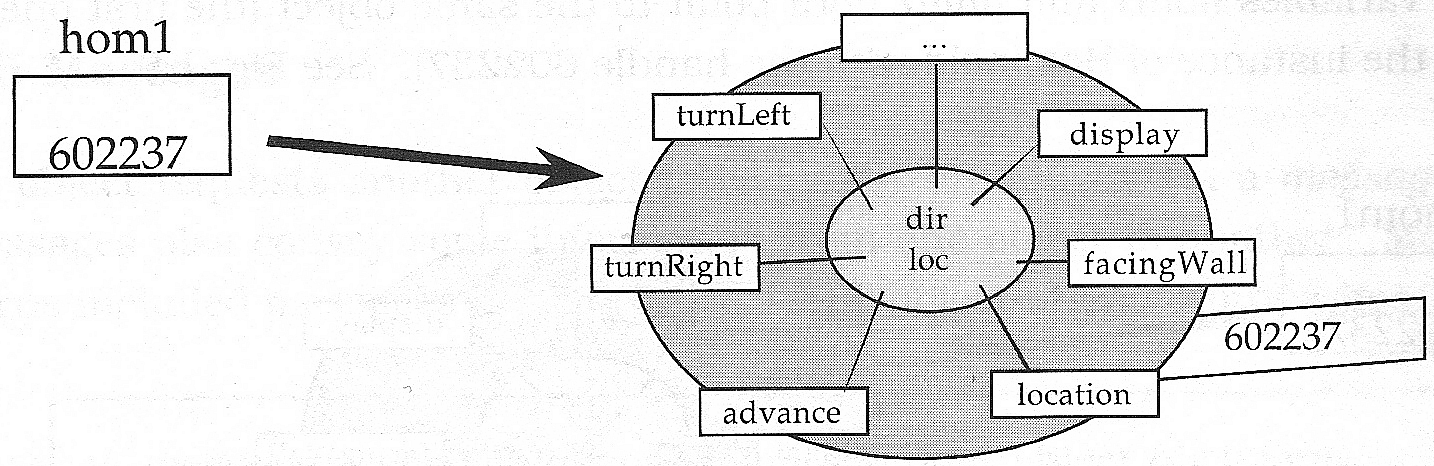
Some OO languages (C++ for example) use the location in memory as a handle (remember pointers?)
Example2: Lets create another object:
var hom2: Hominoid := Hominoid.New; // books notation Hominoid *hom2 = new Hominoid(); // C++ notation
This will create a second object (of class Hominoid) with a new handle (e.g. 142857) and stores that handle in hom2.
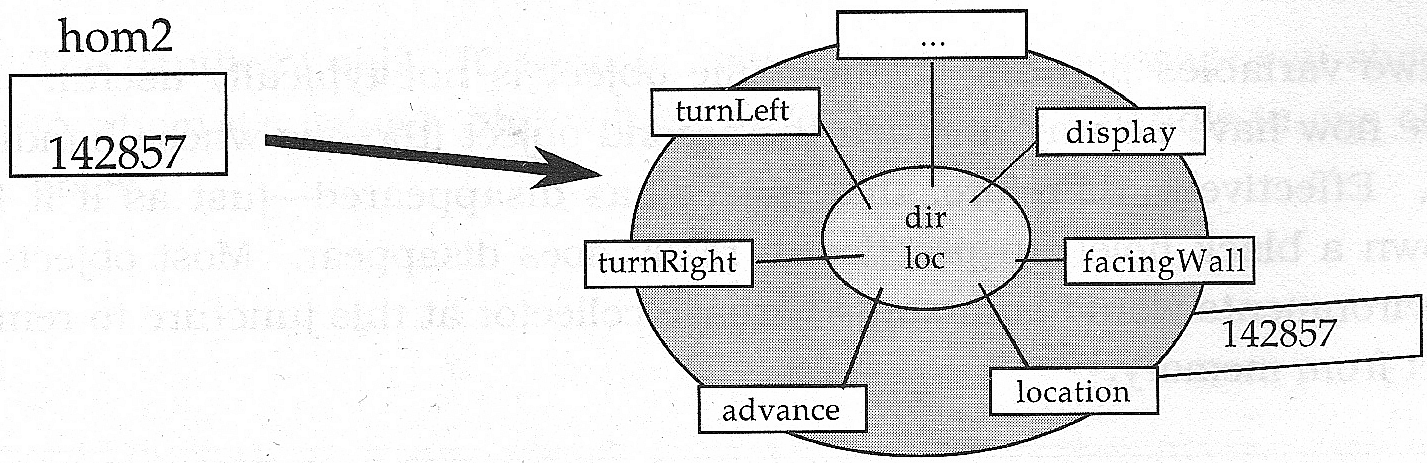
Now what happens after the assignment?
hom2 := hom1; // book hom2 = hom1; // C++
Now both variables hom2 and hom1 hold the same object handle, they both point to the same object!!!
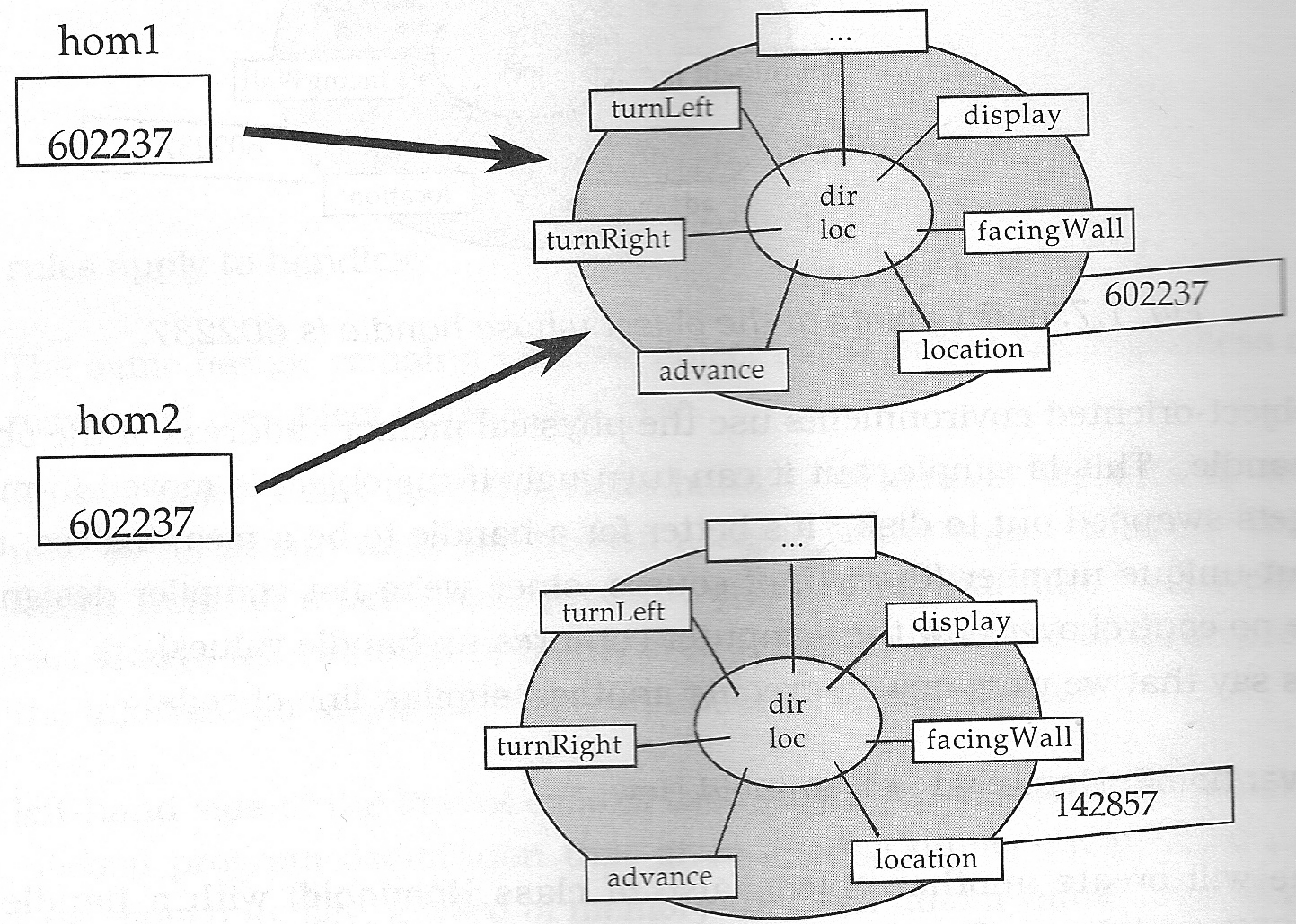
Having two variables pointing at the same object makes very seldom sense.
Even worse: The object at 142857 is now unreachable! We have no variable that holds its handle! That object has now disappeared. In C++: Memory Leak. In Java: Garbage collector.
Practice: which object handles are stored in these variables after this code has executed? Which object(s) is/are "lost" ?
Hominoid *hom1 = new Hominoid(); // assume handle 111 Hominoid *hom2 = new Hominoid(); // assume handle 222 Hominoid *hom3 = new Hominoid(); // assume handle 333 hom3 = hom2; hom2 = hom1;
Solution:
hom3 point to handle 222
hom2 points to handle 111
hom1 points to handle 111
the object at handle 333 is lost
Notation in UML: Similar to class, but different!
Name is underlined to signify instance.
The format for the name is object handle (or variable name), then a colon, then the class, e.g. hom1 : Hominoid
object handle / variable name may be ommited, e.g. : Hominoid (notice the colon is still there). In this case we talk about an "anonymous instance"
Short notation: Just the name and its class in a box
Long notation: shows one additional compartment that lists the attribtues with name, an equal sign and the value, e.g. direction = 180
Instances are shown in an object diagram

This Hominoid has 3 instances:
Two of then are anonymous, one has a name (hom)
All of them have a value for the attribute direction, but it is only shown on one of them
Notes for MagicDraw:
MagicDraw calls the instance attributes "slots"
Slots are not shown by default (must be turned on)
No attribute has a value by default (must go into menu and "create value")
MagicDraw shows classes in orange, instances in yellow ( this is completely optional, the underline is what makes the real difference!)
Practice: Draw two instances for the class Car, as given in this diagram:
A possible solution:
Since every object (must) have an object handle, relations can be shown like other attributes:
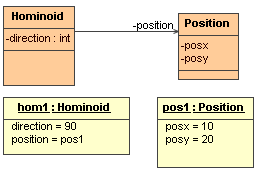
In this case we show the relation Hominoid - Position as attribute (slot), where the value is the object handle of position.
Warning: We use human-readable handles in UML diagrams, but in reality these are the internal object handles (strange numbers that make no sense). The handles shown on UML diagrams do not necessarily need to match the names used for the variables in actual programming!
Assiciations can still be shown, but they have no labels. Rather the information should be shown in the slots
When an attribute has multiple values (e.g. a car has 4 wheels), then the elements are listed separated by a comma.
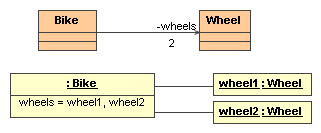
Now draw an object diagram for the modern bomb timer. Use 3 bombs and 1 timer. Add all slots needed (on Timer and Bomb)
A solution: